Cisco Duo Blog
Product & EngineeringMeet the new Duo IAM
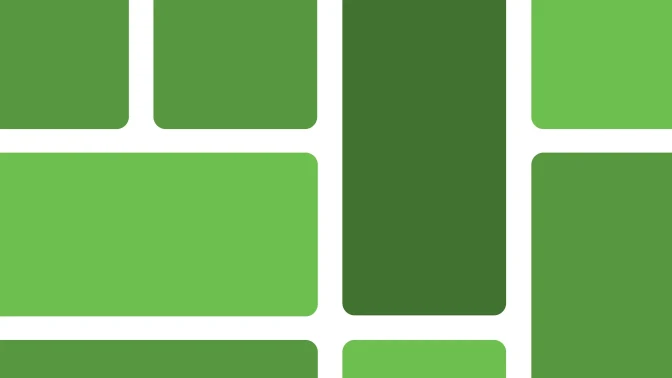
Failing open: A lesson in attention to configurations
Duo’s AI and Security Research team digs into authentication data and finding anomalies that can be searched for, alerted on, or remediated using AI and ML.
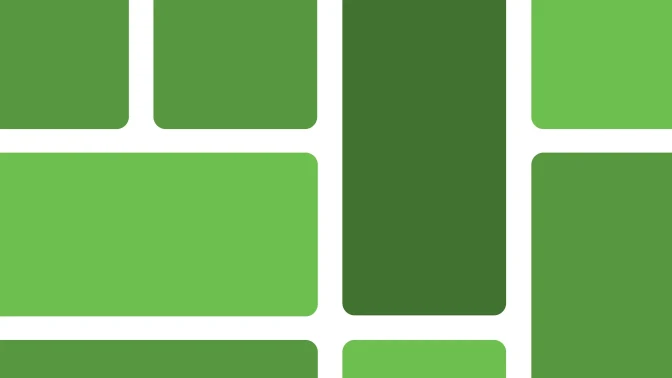
What’s new for you: Duo is now identity and access management
Duo’s security-first approach to Identity and Access Management delivers new capabilities to help you achieve security by default, and usability people love.
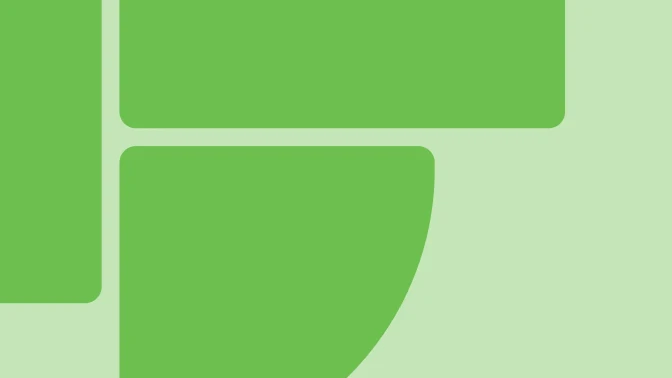
Duo Proximity Verification: Deployable phishing-resistant MFA
With Proximity Verification built into Duo’s IAM solution, organizations get strong, phishing-resistant authentication.
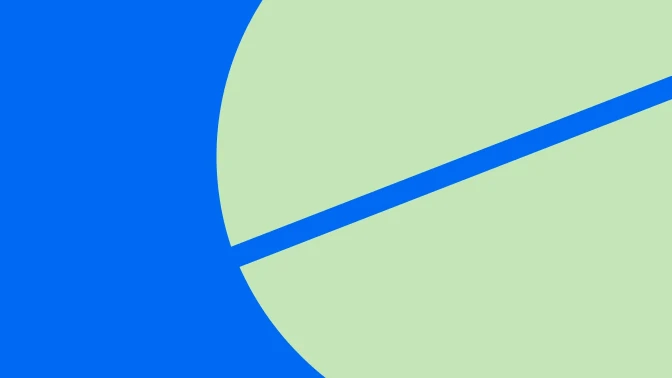
End-to-end phishing resistance that's actually deployable
Attackers have evolved. They are bypassing traditional MFA. To combat this, Duo is making it easy to deploy phishing-resistant MFA end-to-end.
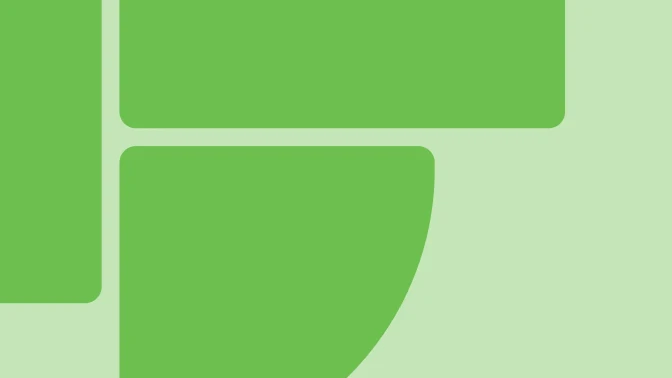
New device? No problem: Enhanced Duo Instant Restore for Android
Duo’s Instant Restore feature is a great way to transfer Duo functionality to a new device. See how updates to Duo Mobile on Android devices make this seamless.
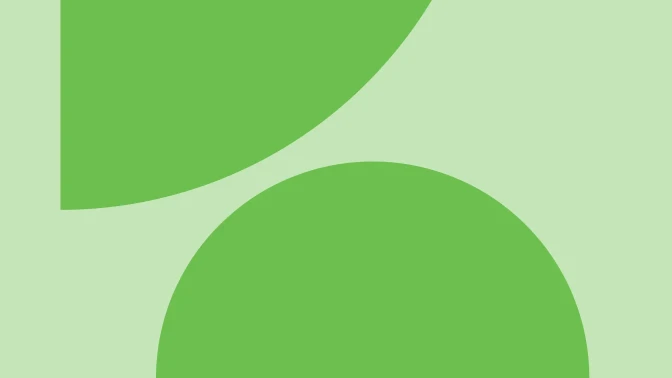
Introducing Cisco AI Assistant for Duo
We’re excited to announce the Cisco AI Assistant for Duo, our newest addition in Cisco’s suite of AI Assistants enhancing the security and IT team experience.
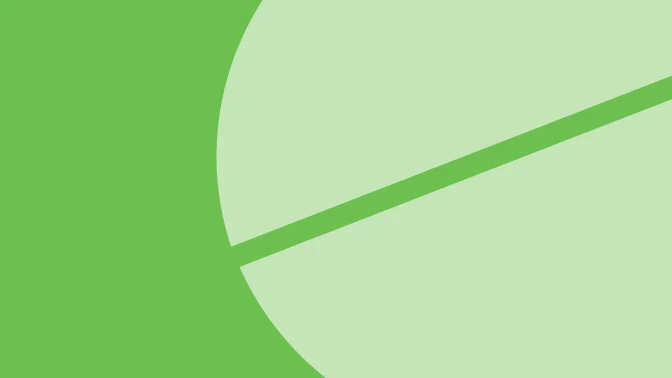
Why a security-first approach to IAM matters more than ever
Security-first means putting security first whenever possible. Duo puts security controls on by default, not as a costly or incomplete add-on.
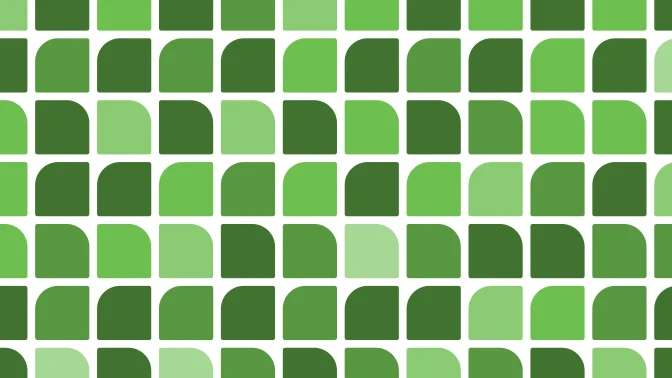
Come see Duo at Identiverse 2025
Duo dramatically expands offering with new directory and phishing-resistant functionality to address the sharp rise in identity-based attacks.
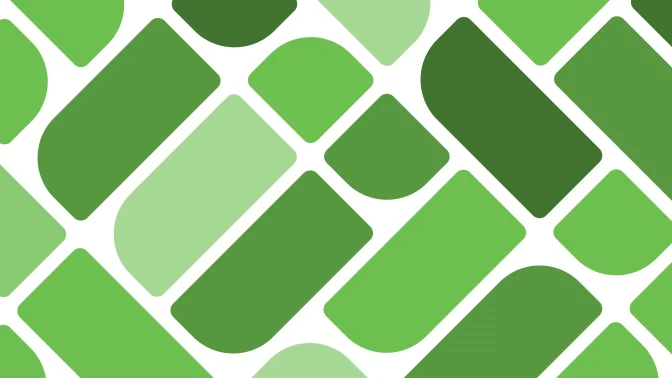
No Agent Required: Duo & Microsoft Edge for Business improve device trust
Restrict access to only trusted devices using Duo Trusted Endpoints and the managed Microsoft Edge for Business browser without installing an endpoint agent.
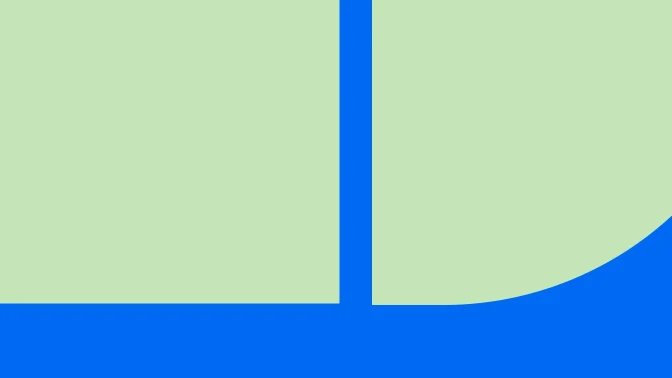
Introducing Duo Wear: Seamless MFA from your wrist!
Learn more about Duo Wear, a companion app for Duo Mobile that brings fast and easy multi-factor authentication to your Wear OS smartwatch!